#
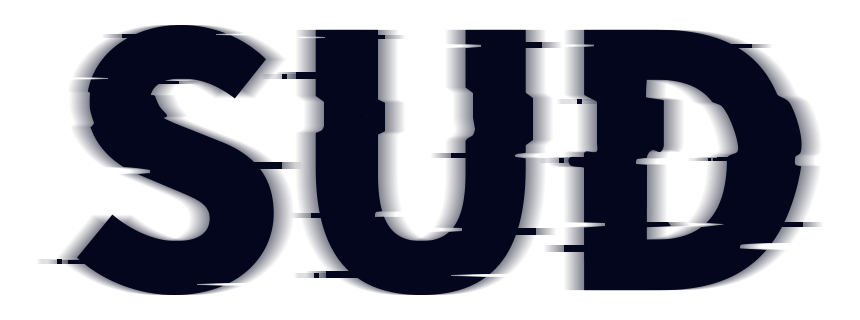
Description
- Before requesting the server-side API, you need to first request the current interface to obtain the actual URL address of the server-side API.
- Obtain the server-side API configuration file using app_id and app_secret. It is recommended to pull this from the server and cache it locally (you can schedule regular pulls of the API address, suggesting an interval of one day or longer, or refresh when access fails. If the refresh fails, use the previous cached result and retry).
Request URL
App service signature implementation method
- The app service signature is generated based on the HMAC algorithm, with the
app_secret
as the password, and the app_id
as the data for encryption.
Java example code:
import cn.hutool.crypto.digest.HMac;
import cn.hutool.crypto.digest.HmacAlgorithm;
class Test {
public static void main(String[] args) {
String data = "appId";
byte[] key = "appSecret".getBytes();
HMac mac = new HMac(HmacAlgorithm.HmacMD5, key);
String appServerSign = mac.digestHex(data);
System.out.println(appServerSign);
}
}
Go example code
package main
import (
"crypto/hmac"
"crypto/md5"
"encoding/hex"
"fmt"
)
func main() {
key := "appSecret"
data := "appId"
hmac := hmac.New(md5.New, []byte(key))
hmac.Write([]byte(data))
fmt.Println(hex.EncodeToString(hmac.Sum([]byte(""))))
}
Node example code
const Crypto = require('crypto');
function main() {
let key = 'appSecret';
let data = 'appId';
let hmac = Crypto.createHmac('md5', key);
let sign = hmac.update(data).digest('hex');
console.log(sign);
}
Request Method
- Transmission protocol: HTTPS
- Request method: GET
Content-Type: application/json
needs to be added in the header.
Request Parameters
None
Request Example
Response Parameters
Server-side API
Parameter Name |
Type |
Description |
mg_list |
string |
Interface address for obtaining the game list (used by the server) |
mg_info |
string |
Interface address for obtaining game information (used by the server) |
get_game_report_info |
string |
Interface address for querying game round information (used by the server) |
get_game_report_info_page |
string |
Interface address for paging query of game round information (used by the server) |
query_game_report_info |
string |
Interface address for querying game reporting information based on custom game round ID (used by the server) |
get_player_results |
string |
Obtain player game results (used by the server) |
report_game_round_bill |
string |
Report the consumption of currency for each round of the game (used by the server) |
push_event |
string |
Push events to the game server (used by the server) |
create_order |
string |
Create order (used by the server) |
query_order |
string |
Query order (used by the server) |
query_match_base |
string |
Query basic information of a single game (used by the server) |
query_match_round_ids |
string |
Query all round IDs in a single game (used by the server) |
query_user_settle |
string |
Query user settlement information (used by the server) |
auth_app_list |
string |
Query authorized application list (used by the server) |
auth_room_list |
string |
Query authorized room list (used by the server) |
Matching API
Parameter Name |
Type |
Description |
create_match |
string |
Initiate matching (used by the server) |
cancel_match |
string |
Cancel matching (used by the server) |
query_game_config |
string |
Query matching game mode configuration (used by the server) |
query_user_matching |
string |
Query user matching information (used by the server) |
Bullet Screen API
Parameter |
Type |
Description |
init |
string |
Initialization (used by the server) |
command |
string |
Send command (used by the server) |
refresh |
string |
Refresh (used by the server) |
Web3 API
Parameter Name |
Type |
Description |
get_nft_details |
string |
Interface address for obtaining the details of the worn NFT (used by the server) |
refresh_details |
string |
Interface address for refreshing the token of the worn NFT details (used by the server) |
Response Example
{
"api": {
"get_mg_list": "",
"get_mg_info": "",
"get_game_report_info": "",
"get_game_report_info_page": "",
"query_game_report_info": "",
"report_game_round_bill": "",
"push_event": "",
"auth_app_list": "",
"auth_room_list": ""
},
"match_api": {
"create_match": "",
"cancel_match": "",
"query_game_config": "",
"query_user_matching": ""
},
"cross_app_api": {
"auth_app_list": "",
"auth_room_list": ""
},
"bullet_api": {
"init": "",
"command": "",
"refresh": ""
},
"web3_api": {
"get_nft_details": "",
"refresh_details": ""
}
}